This Dot Blog
This Dot provides teams with technical leaders who bring deep knowledge of the web platform. We help teams set new standards, and deliver results predictably.
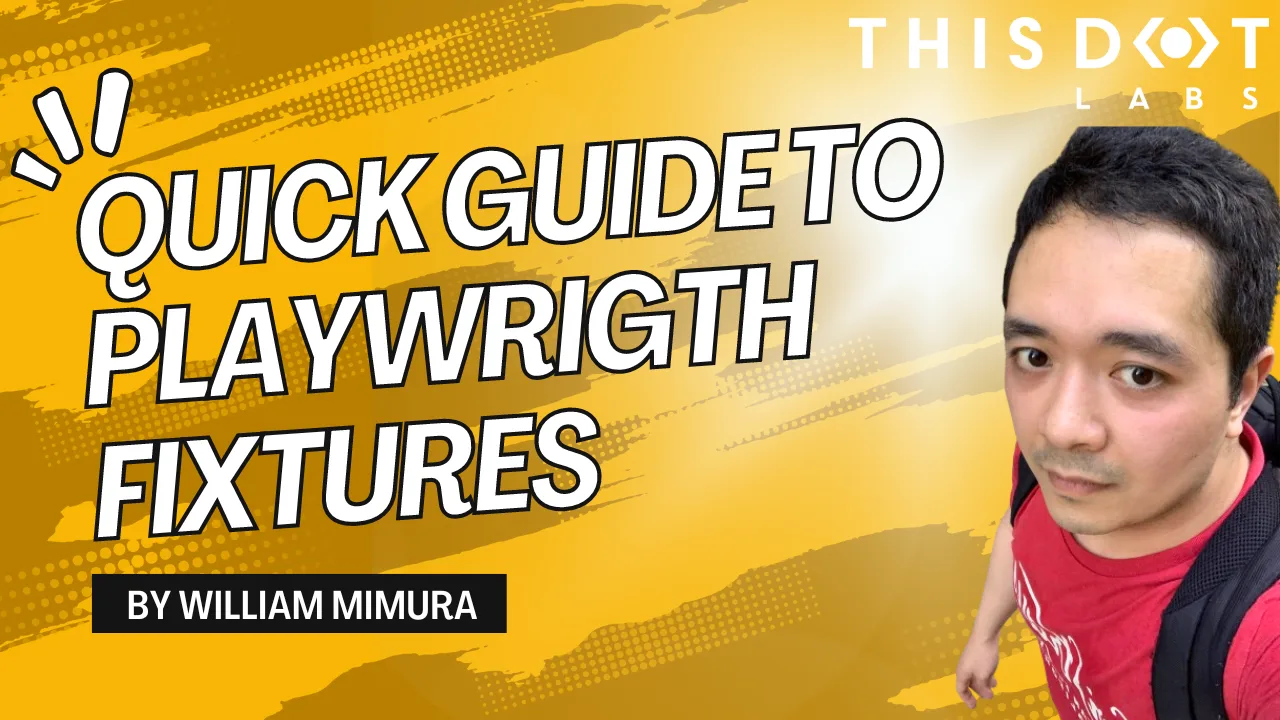
Quick Guide to Playwright Fixtures: Enhancing Your Tests
Explore the essentials of using Playwright fixtures in our latest guide. Learn how to create, implement, and manage fixtures to streamline your end-to-end testing process. This quick guide provides practical examples....
Jul 26, 2024
2 mins
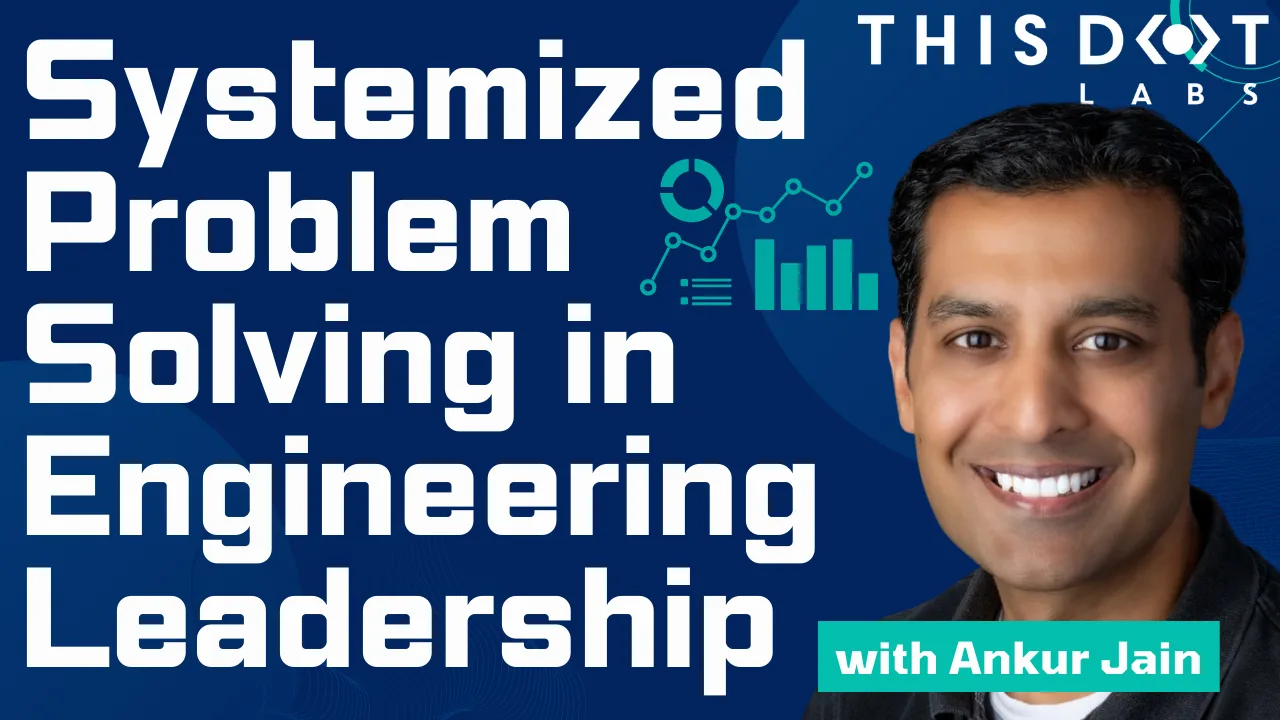
Systemized Problem Solving in Engineering Leadership Using Data with Ankur Jain
What is it like to transition from technologies to Fractional CTO? How much do systems matter when operating at the C Level? Ankur Jain, Fractional CTO and Founder at Sprout discusses the transition from being a technologist to a fractional CTO, and how to define and meet engineering KPIs. He emphasizes the significance of systemizing and design thinking in problem-solving, stressing the need to understand customer needs and deliver effective solutions. By adopting a systematic approach, businesses can effectively identify and address customer needs. Design thinking, on the other hand, encourages a human-centered approach to innovation, ensuring that technology solutions are not only functional but also user-friendly. Ankur insights remind us that successful technology implementation requires a deep understanding of customer pain points and a commitment to delivering effective solutions. In an era where data is abundant, Ankur emphasizes the value of making data-driven decisions. However, he cautions against relying on biased data, which can lead to flawed conclusions. He advises businesses to carefully analyze and interpret data, ensuring that it aligns with the goals and objectives of the organization. By leveraging data effectively, businesses can gain valuable insights, make informed decisions, and drive growth. Ankur highlights the significance of ensuring product-market fit by closely collaborating with early customers. By actively involving customers in the development process, businesses can gain valuable feedback and insights, ensuring that their products or services meet the needs of the target market. Ankur's emphasis on customer collaboration serves as a reminder that successful technology implementation requires a customer-centric approach, where the end-users' needs and preferences are at the forefront of decision-making. Ankur advocates for mentorship and continuous learning in leadership roles. He emphasizes the value of seeking guidance from experienced professionals and gradually growing within organizations. His insights remind us that leadership is a journey of growth and development, and that embracing mentorship and continuous learning can help individuals navigate the complexities of technology leadership more effectively. Download this episode here....
Jul 26, 2024
2 mins
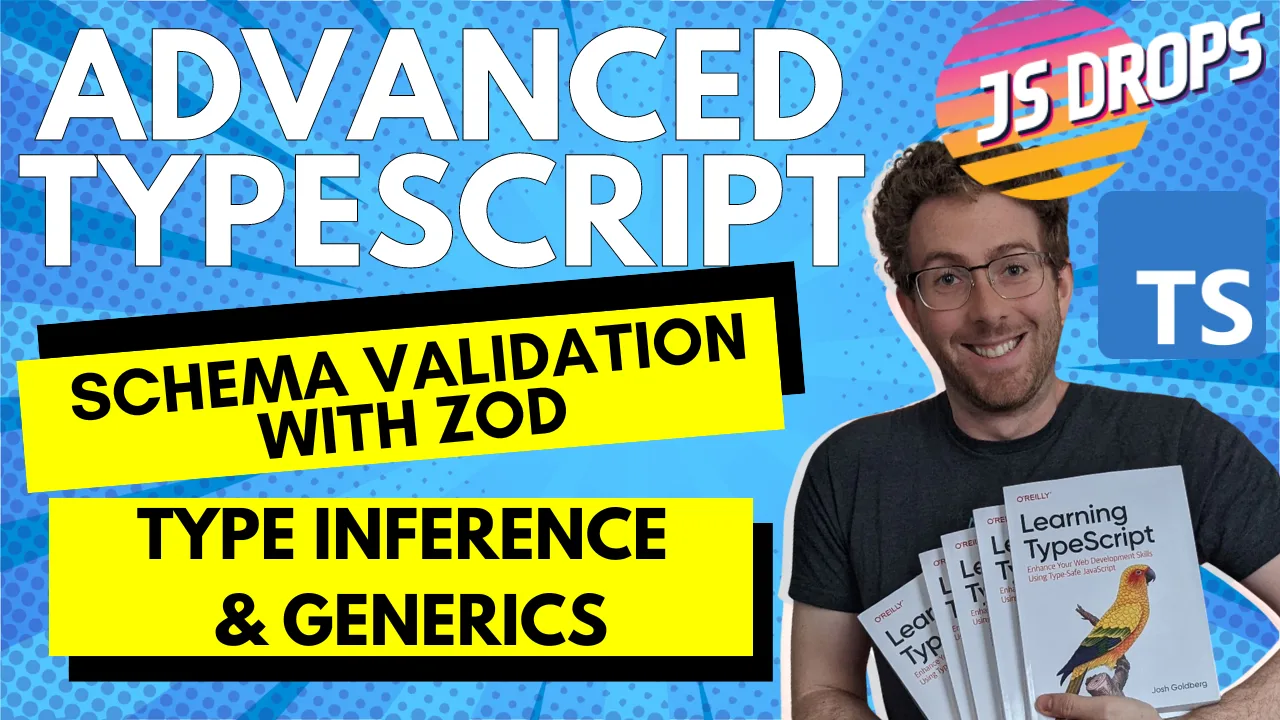
Advanced TypeScript - Schema Validation with Zod - Type Inference & Generics with Josh Goldberg
In this episode of Modern Web, Josh Goldberg discusses the benefits of TypeScript ESLint v8 and as well as other various topics related to JavaScript tools, AI in coding, and industry dynamics. Josh breaks down the latest version of TypeScript ESLint, v8. He points out the big performance boosts and introduces the cool new feature of type-aware linting. With this, developers can catch potential errors and follow best practices by using TypeScript's static type checking. This not only cuts down on bugs but also makes the code easier to read and maintain. Tracy and Josh talk about the importance of using the right tools for better coding results. They discuss how the Gartner hype cycle can influence developers' choices and warn against adopting tools just because they’re trendy. Instead, they suggest carefully evaluating tools based on specific needs and project requirements. By picking the right tools, developers can simplify their workflows, improve code quality, and get better outcomes overall. The conversation also touches on the impact of companies like Vercel and the unexpected consequences in tech development. While new tools and technologies can be super beneficial, they can also bring unexpected challenges. It’s important for developers to be aware of these potential issues and address them to ensure smooth development and successful projects. They also chat about the "trough of disillusionment" in tech adoption and mention upcoming typed linting tools which aim to further improve code quality and developer productivity. Lastly, the two talk about the SquiggleConf conference, which focuses on web development tools. They explain the term "squiggle" in error indicators and why clear, informative error messages are important for helping developers debug and troubleshoot. The conference is a great place for developers to learn about the latest web development tools and share tips on improving the developer experience. Check it out https://2024.squiggleconf.com/ on October 3-4, 2024 in Boston, MA!...
Jul 26, 2024
2 mins
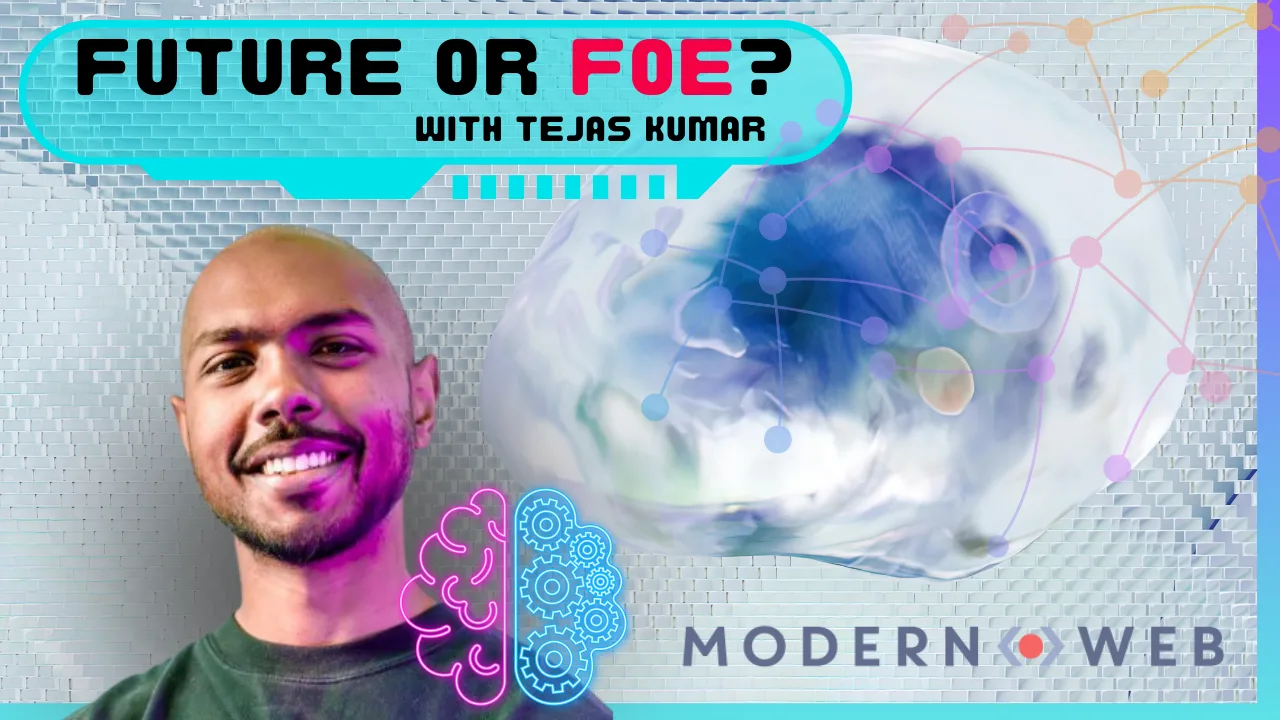
Agentic AI: What Does AI Agency Mean Our Future? Safety and Security with Tejas Kumar
Tejas Kumar and Tracy Lee discuss AI models, tool calling, and Vercel's AI SDK for generating components. They explore AI agency, the importance of documentation, safety concerns, regulation, and the need for human oversight in AI development....
Jul 24, 2024
1 min
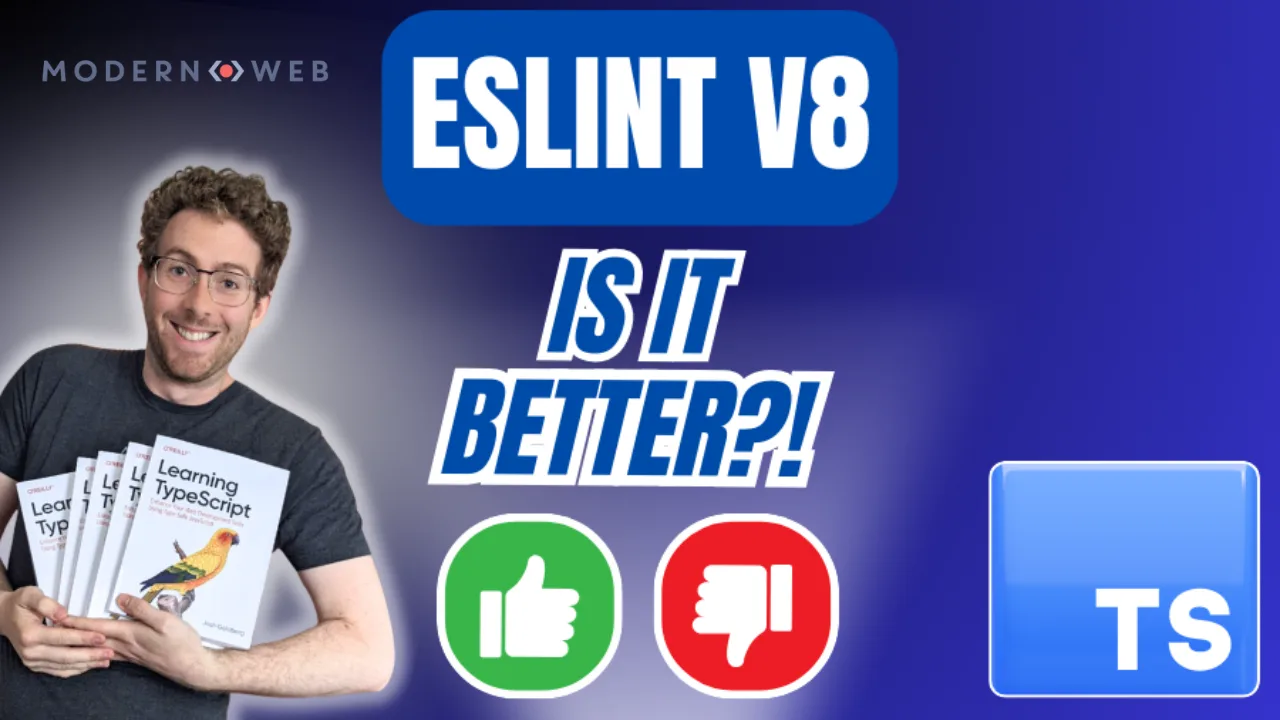
What’s Great About TypeScript ESLint v8 + The “Trough of Disillusionment” in Adoption with Josh Goldberg
In this episode of Modern Web, Josh Goldberg discusses the benefits of TypeScript ESLint v8 and as well as other various topics related to JavaScript tools, AI in coding, and industry dynamics. Josh breaks down the latest version of TypeScript ESLint, v8. He points out the big performance boosts and introduces the cool new feature of type-aware linting. With this, developers can catch potential errors and follow best practices by using TypeScript's static type checking. This not only cuts down on bugs but also makes the code easier to read and maintain. Tracy and Josh talk about the importance of using the right tools for better coding results. They discuss how the Gartner hype cycle can influence developers' choices and warn against adopting tools just because they’re trendy. Instead, they suggest carefully evaluating tools based on specific needs and project requirements. By picking the right tools, developers can simplify their workflows, improve code quality, and get better outcomes overall. The conversation also touches on the impact of companies like Vercel and the unexpected consequences in tech development. While new tools and technologies can be super beneficial, they can also bring unexpected challenges. It’s important for developers to be aware of these potential issues and address them to ensure smooth development and successful projects. They also chat about the "trough of disillusionment" in tech adoption and mention upcoming typed linting tools which aim to further improve code quality and developer productivity. Lastly, the two talk about the SquiggleConf conference, which focuses on web development tools. They explain the term "squiggle" in error indicators and why clear, informative error messages are important for helping developers debug and troubleshoot. The conference is a great place for developers to learn about the latest web development tools and share tips on improving the developer experience. Check it out on October 3-4, 2024 in Boston, MA! Download this episode here....
Jul 19, 2024
2 mins
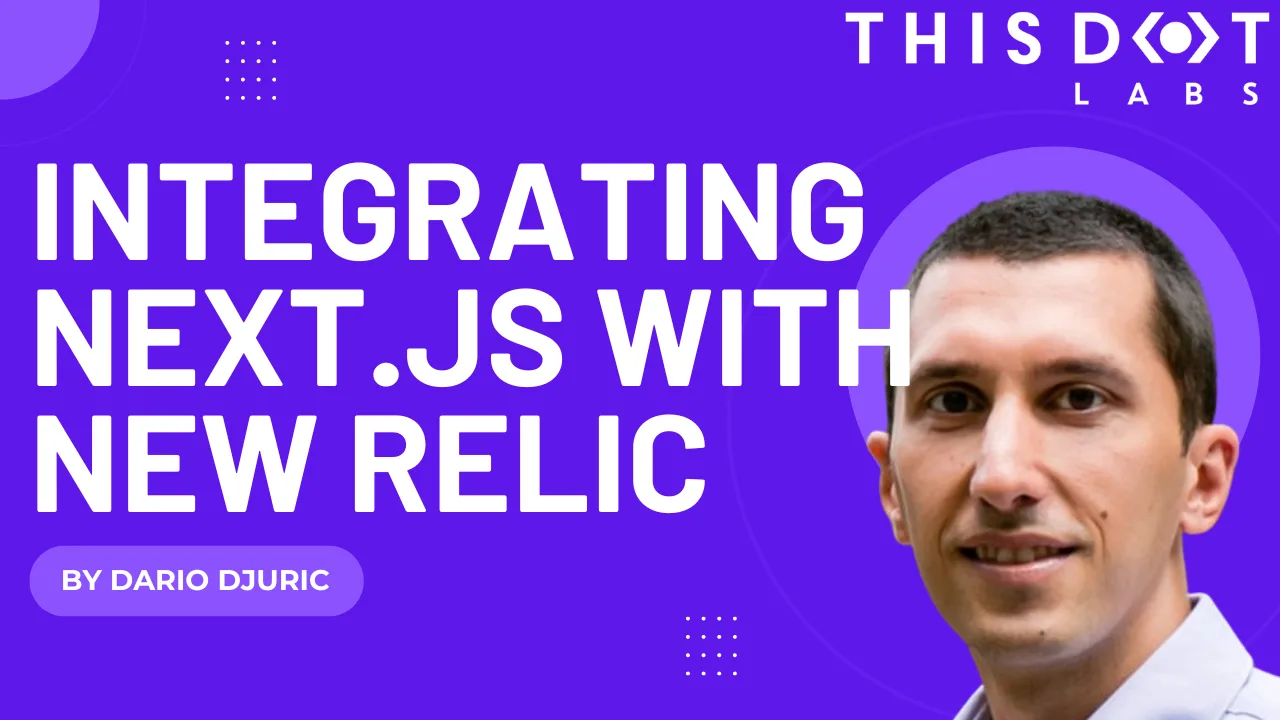
Integrating Next.js with New Relic
Check out our detailed guide on integrating New Relic’s application monitoring with Next.js....
Jul 19, 2024
7 mins
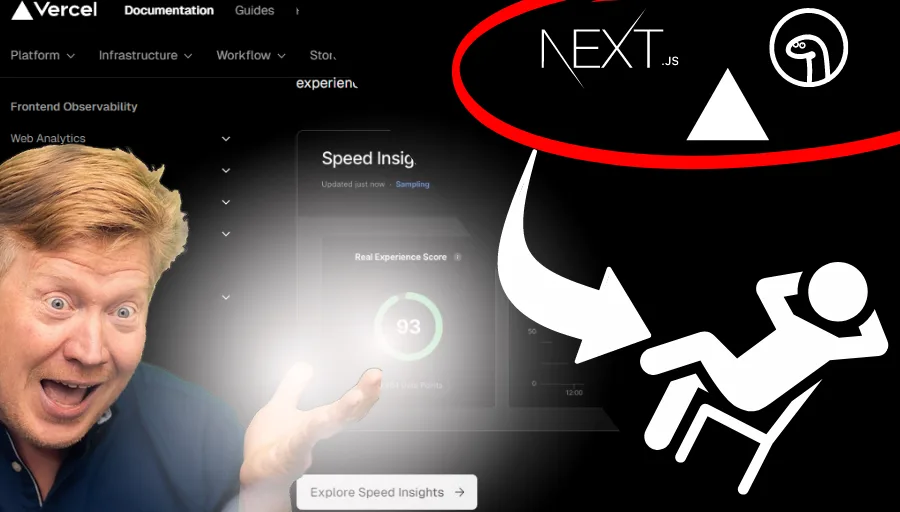
These JavaScript Tools Make Collaboration and Deployment Easier with Jack Herrington
Join Jack Herrington and Tracy Lee at CascadiaJS 2024 as they talk about content creation, experimenting with new tools, and continuous learning. They cover some of the latest in what’s going on in the Vercel, Next.js, and Deno Deploy ecosystems, and what these teams are doing to enable easy deployment and better community collaboration. Tracy and Jack's discussion highlights the lack of educational resources on advanced web development topics. This scarcity poses an opportunity for developers who want to showcase their expertise in areas like app router and React server components. By actively engaging in knowledge sharing, developers can demonstrate their deep understanding of these complex concepts and set themselves apart from the competition. Jack emphasized Vercel's role in simplifying deployment with its easy commands and Next.js integration for seamless collaboration and performance. Next.js was highlighted for its advanced features and simplified development process, including efficient routing and SEO benefits. Deno Deploy, built on Deno, offers serverless deployment without traditional infrastructure management, focusing on code writing and scalability. Throughout, the importance of community and knowledge sharing in platforms like Vercel, Next.js, and Deno Deploy was underscored, enabling developers to share, receive feedback, and stay updated with advancements. Download this episode here....
Jul 18, 2024
1 min
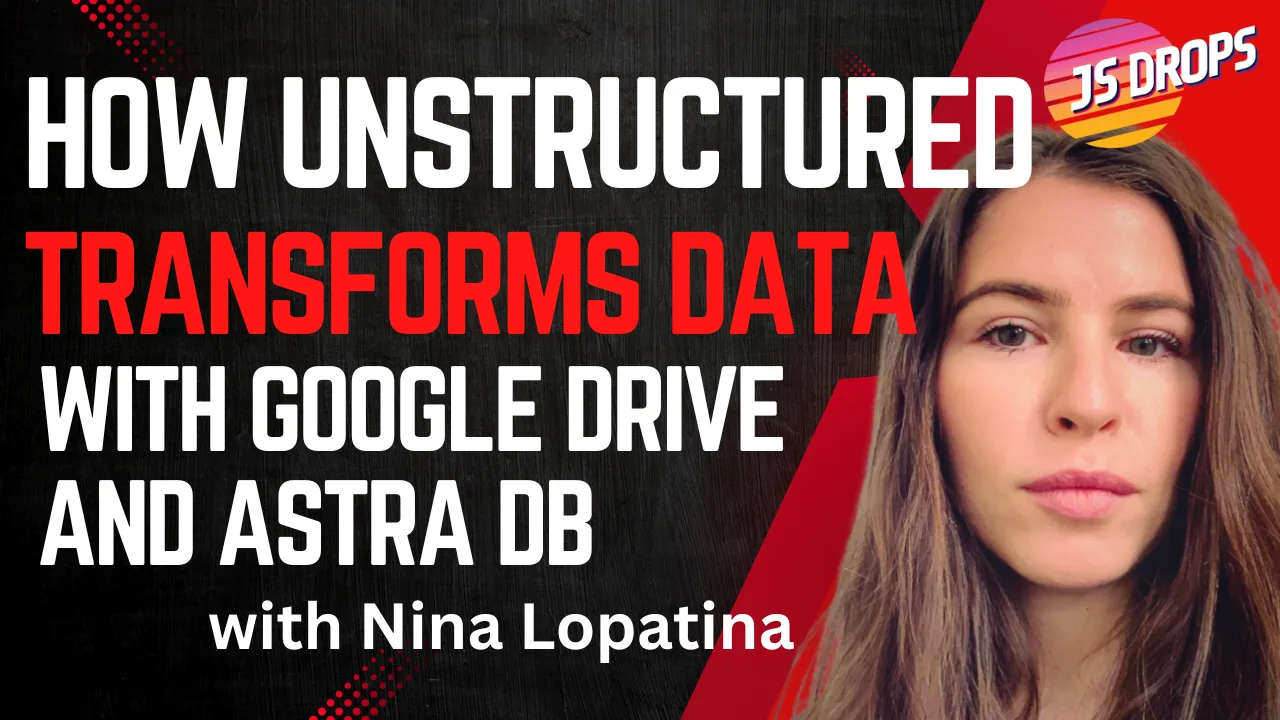
How Unstructured Transforms Data with Google Drive and Astra DB with Nina Lopatina
Unstructured data lacks a predefined format, posing challenges for machine understanding. However, companies like Unstructured offer solutions to overcome this hurdle. This JSDrops training hosted by Nina Lopatina explores how Unstructured utilizes tools such as Google Drive and Astra DB to convert unstructured data into machine-readable formats, opening new possibilities for businesses and individuals. Nina reviews the setup process, discussing the critical roles of API keys and service accounts. API keys secure access to Google Drive, while service accounts facilitate interactions with Astra DB. This setup ensures seamless data access and processing, establishing a robust foundation for Unstructured's operations. She explains how to set up an efficient notebook environment, focusing on library installations, Google Drive connectivity, and security configurations. Emphasizing collaboration, this setup ensures seamless teamwork, enabling Unstructured to efficiently structure and analyze data. During setup, viewers learn to execute code in Google Colab, a cloud-based platform. Troubleshooting API keys underscores the importance of proper configuration for smooth data processing....
Jul 16, 2024
1 min
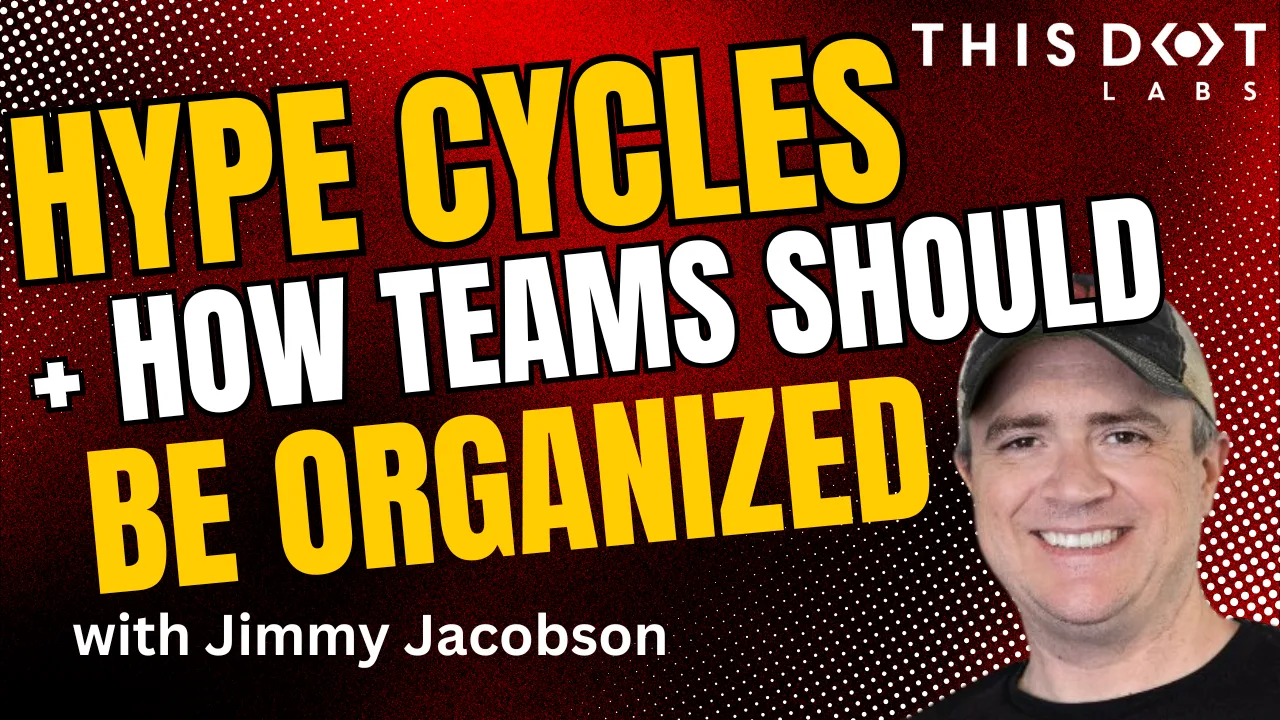
Hype Cycles and How Teams Should Be Organized with Jimmy Jacobson, CTO at Codingscape
Jimmy Jacobson, CTO at Codingscape, sits down with Tracy Lee to discuss engineering consultancy management and team organization. They talk about professional development, hype cycles, and the benefit of investing in social media and marketing both as an organization and as an individual. Both Jimmy and Tracy stress the importance of a flat team structure, which fosters professional development and encourages collaboration among team members. As technology continues to evolve, so do business practices. It is crucial for engineering leaders to adapt and embrace new technologies and methodologies. Tracy Lee and Jimmy Jacobson's podcast provides valuable insights into the world of engineering leadership, technology, and business. From the importance of hiring experienced engineers and product managers to the evolution of business practices, their discussions shed light on the strategies and approaches that drive success. Download this episode here....
Jul 15, 2024
1 min
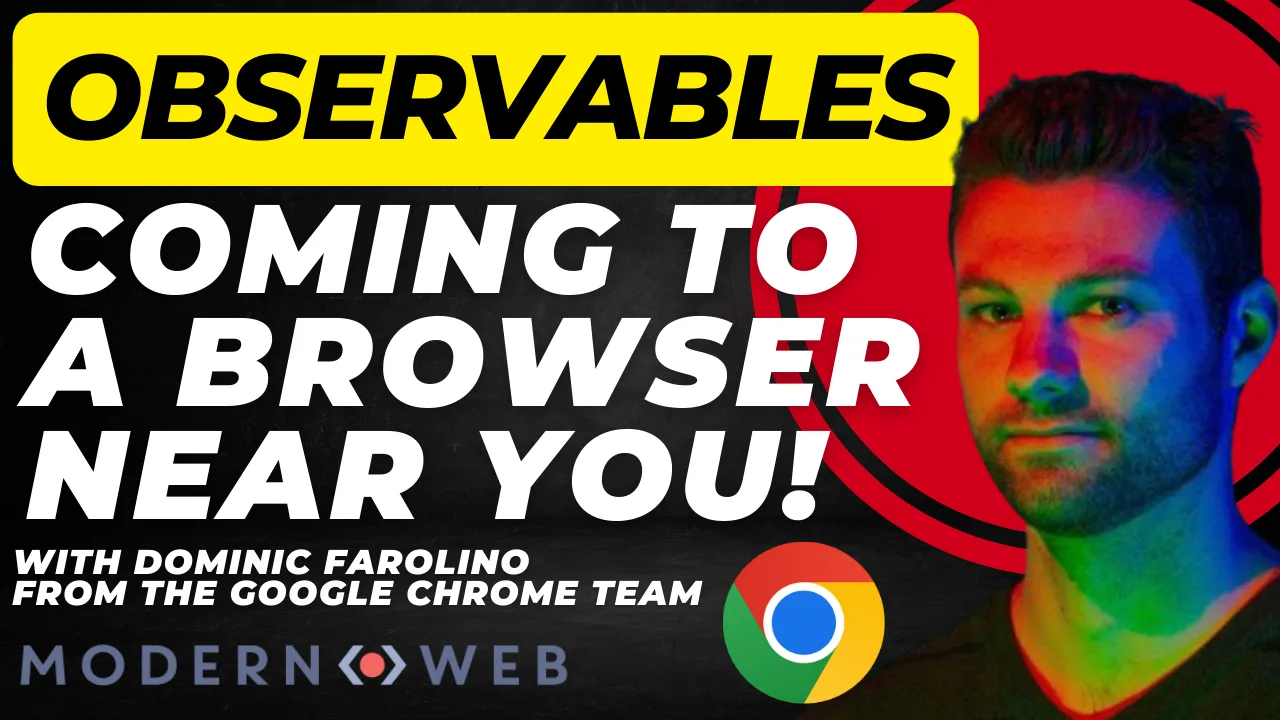
Observables: Coming to a Browser Near You with Dominic Farolino (Google Chrome)
Dominic Farolino, a software engineer on the Chrome team, discusses his involvement in adding observables to the web platform. By incorporating observables into browsers, developers can simplify their workflows and streamline complex APIs (like the Resize Observer). This not only will saves time and effort but also allows developers to focus on creating exceptional user experiences. Adding observables to the browser will drastically affect RxJS. RxJS is a popular library for reactive programming, and integrating observables into browsers will enable developers to handle asynchronous events and data streams more efficiently. This integration opens up a world of possibilities for creating responsive and interactive web applications. This does not mean RxJS will go away, but it will become more like LoDash for events. The incorporation of observables into browsers brings several benefits for web developers. Firstly, it simplifies complex APIs, making them more intuitive and easier to use. This allows developers to write cleaner and more maintainable code. Secondly, by leveraging observables, developers can handle asynchronous events and data streams more effectively, resulting in improved performance and responsiveness. Lastly, it will empower developers with a powerful toolset for reactive programming, enabling them to build more sophisticated and interactive web applications. The conversation in the podcast highlights the collaborative nature of advancing web technologies. Dominic discusses the importance of setting deadlines, sharing updates, and working together to achieve their goals. He expresses optimism about the progress of the project, with positive feedback from WebKit and plans for a future release. This collaborative approach ensures that the enhancements made to web performance benefit developers and users alike. Download this episode here....
Jul 12, 2024
2 mins
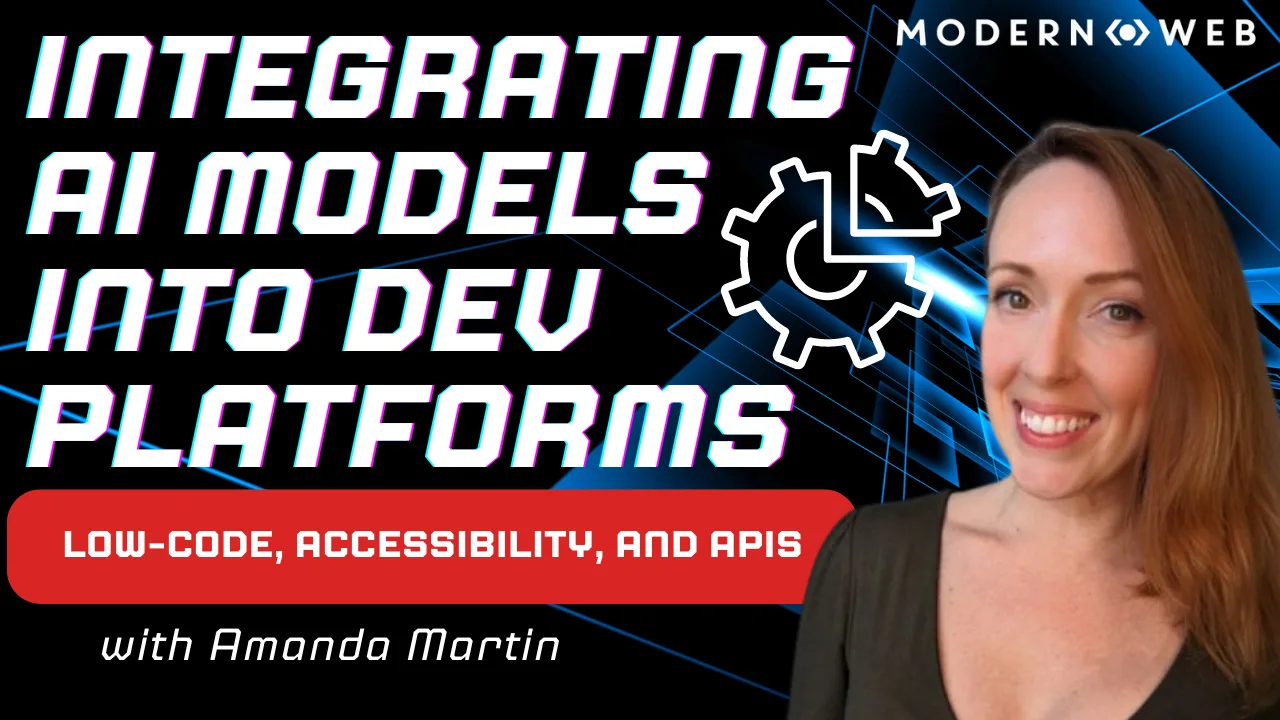
Integrating AI models into Dev Platforms (Low-Code, Accessibility, and APIs) with Amanda Martin from Wix
In this interview at RenderATL 2024, Tracy Lee and Rob Ocel interview Amanda Martin, a developer advocate at Wix, about integrating AI models into web development platforms. The conversation covers incorporating AI into low-code environments, emphasizing the accessibility of AI technologies through APIs and pre-built models. Amanda stresses the importance of understanding tools, security, and reliability, as well as practical applications and inspiring creativity in developers. The conversation touches on the accessibility of AI technologies in low-code environments. Amanda highlights the ease of incorporating AI through APIs and pre-built models, making it more accessible for developers of all skill levels. Understanding the available tools and services is crucial, with considerations for factors like security and reliability. By staying informed and up-to-date, developers can leverage AI to enhance their web development projects. Amanda stresses the importance of showcasing practical applications and inspiring creativity in developers. Rather than simply selling products, she believes in driving meaningful engagement with technology through hands-on experience and genuine passion. By encouraging developers to explore the possibilities of AI, Amanda believes they can unlock their full potential and create innovative solutions. Download this episode here....
Jul 11, 2024
1 min
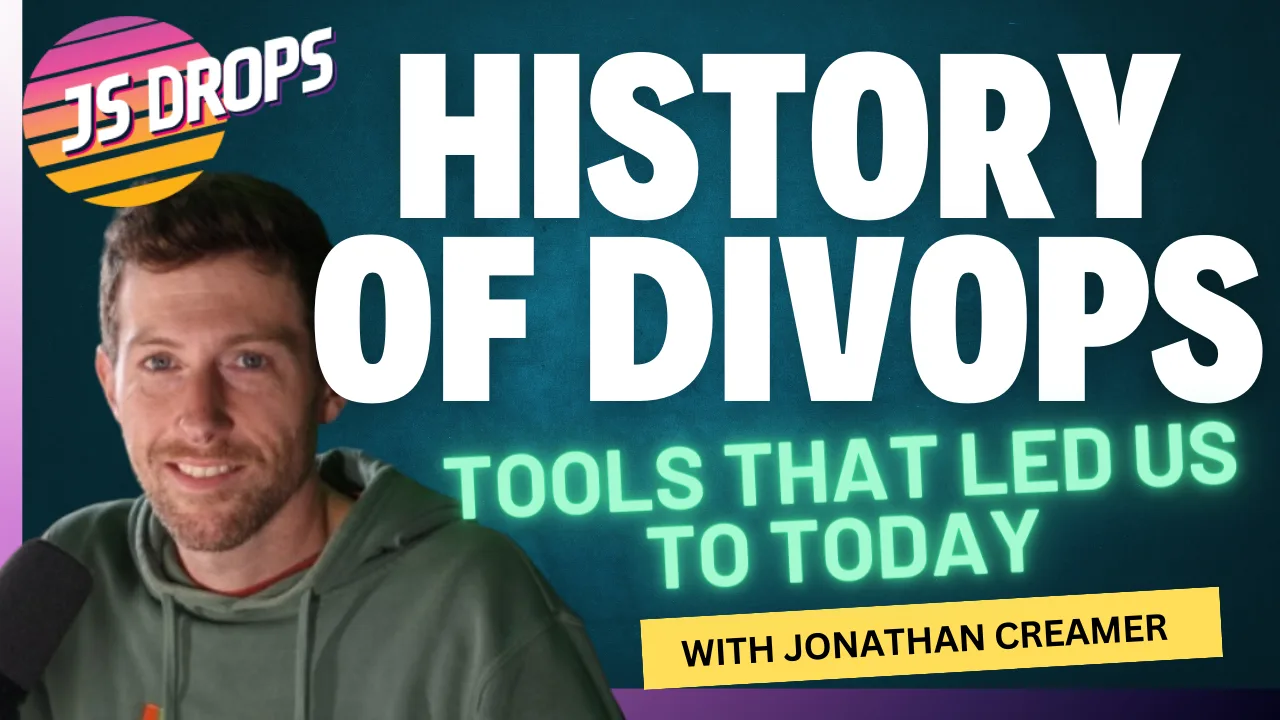
The History of DivOps: The Tools that Led Us to Today
In this JS Drop, Jonathan Creamer, Senior Software Engineer at Microsoft, shares key insights on the history of DivOPs tooling, starting in the days before advancements like Webpack bundles. Jonathan discusses the emergence of bundlers like Webpack and the role of Abstract Syntax Trees (ASTs) in modern web development. Bundlers have revolutionized code organization by allowing developers to modularize their code, enhancing maintainability and scalability. ASTs enable bundlers to efficiently analyze and transform code, optimizing the development process. In the early days of web development, developers had to manually order their scripts to ensure proper dependencies. However, the introduction of tools like AMD and RequireJS transformed the landscape by enabling modular code organization. This shift empowered developers to focus on writing modular code, making their projects more manageable and adaptable to changes. Efficient code management and build automation are vital aspects of modern web development. The podcast highlights the continuous innovation in build tools such as Grunt, Gulp, Vite, and Parcel. These tools automate repetitive tasks, optimize code, and streamline the development workflow, allowing developers to save valuable time and effort....
Jul 10, 2024
1 min