There are a few posts that teach you how to develop Angular applications but some of them are missing some of the tips that I'm going to mention here.
FYI: some of the tips can be used in any kind of enterprise app, not just angular.
I'm not going to get into detail on how to apply each one of the tips or tools. I will only talk about them overall for you to start applying them in your Angular enterprise project.
Remember that all tips mentioned are not mandatory but will help your team to write cleaner, better, and more scalable application.
Content Structure
-Typing -SASS -State Management -Libraries -Monorepos -NPM for Enterprise -Lazy Loading -Components -Component Libraries -Reactive Programming with RxJS -Compilation -Design Systems -Angular with Bazel -VS Code Tools
Typing
Typing with TypeScript
When working on such a big application, like an enterprise application, with a big number of developers, typing your code is very important. This will help the developers in preventing mistakes and understanding the code base faster.
so... stop using any
Please, stop it!
Typescript Entities -classes -enums -interfaces (and types)
DON'T DO:
tasks$: any;
DO:
tasks$: Observable<Task[]>;
SASS
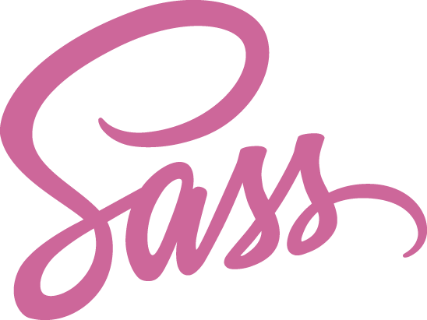
Time to power up your CSS with SASS. By using SASS, you are going to be more DRY which means you won't be repeating yourself. Your CSS code will be more structured, cleaner, and more readable.
When creating a new Angular project using the CLI, it will ask you "what stylesheet format would you like to use?", select SCSS.
If you want to get started with SASS, visit the official docs:
State management
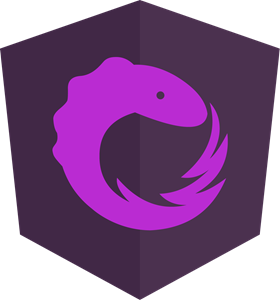
It is very important to handle the state of your application. By handling the state of your app, you will gain some of the benefits like:
-Better performance: The way Angular works when using state, the data updates can flow down through your components relying on slices of the store.
-Centralized immutable state: The state living in a central location inside of your app.
-Save time: Imagine you have an application with a very elaborated workflow where you have to write a bunch of data in the forms and click multiple buttons. Thanks to a tool like Redux Dev Tools, it allows you to travel in time in your application so you don't have to do all the workflow again to test your app - you can simply go to a specific point.
-Easy to test:
All state updates are handled in reducers which are pure functions. Pure functions are extremely simple to test, as it is simply input in
and assert against output
.
A lot of people don't like using state management tools, so they decide to just use RxJS and services. If you have a very good data flow you may not need to handle the state with some of the tools previously mentioned, but you essentially are going to be inventing the wheel. If you are going to do that, you might as well use a tool that is used by millions of developers.
Libraries
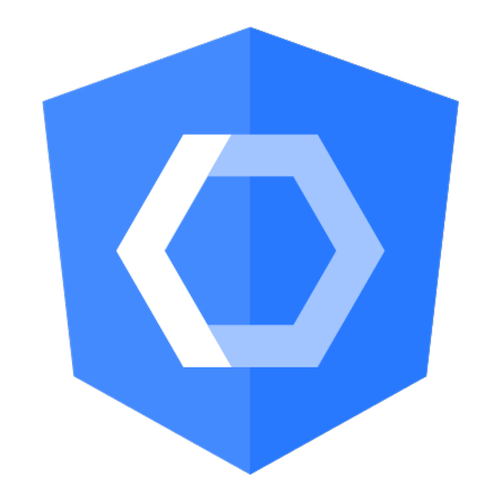
If you are working in a big company the probability of you need to share multiple pieces of code is probably very high, so why would you make your engineers rewrite existing code? Thanks to Angular libraries you can avoid this by creating a sharable code like components, services, etc across your organization. Libraries will allow your apps to avoid code inconsistencies and different standards and will help your company to save money.
How to create a library? https://medium.com/@tomsu/how-to-build-a-library-for-angular-apps-4f9b38b0ed11
NPM for Enterprise
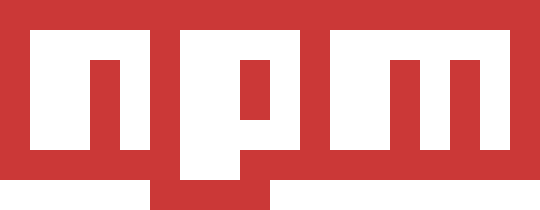
After talking about Angular libraries you may be wondering: how can I share them across my organization?
Well, NPM offers solutions for enterprise applications. Your public npm package will be kept private and only visible/available to your organization.
To learn more about NPM Enterprise visit: https://www.npmjs.com/products/enterprise
Monorepos
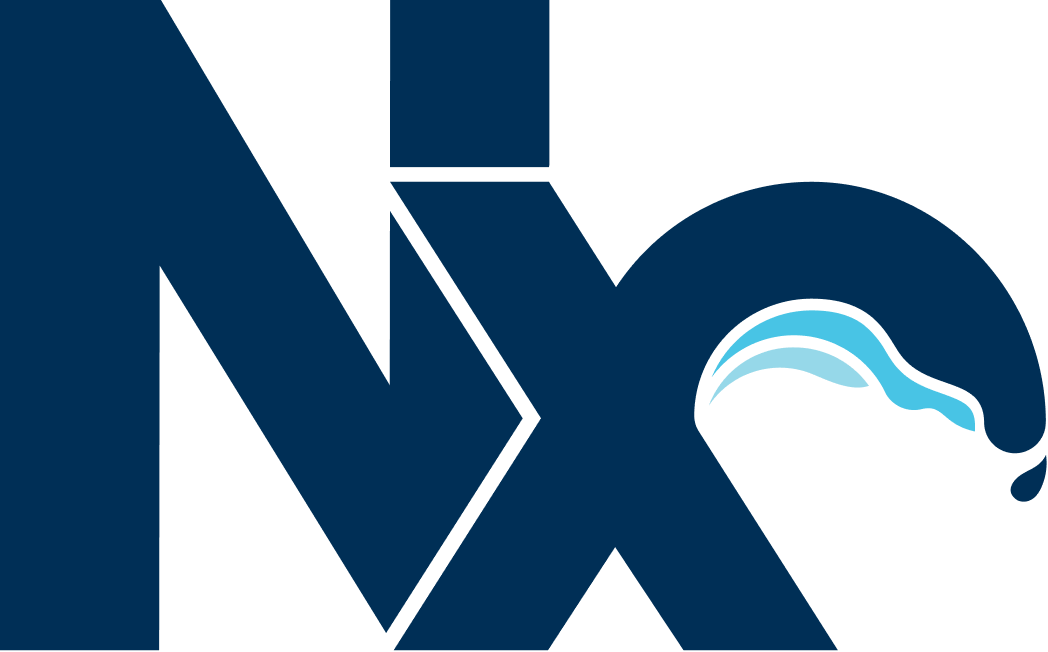
Not everything is pretty with Angular libraries when working on enterprise applications.
-Every time a new change happens in a shared repo, you need to go to all your projects using this library and update their npm packages -Every shared library needs to have its own CI/CD workflow -Each CI/CD workflow should handle versioning of changes -There can be mismatches with different npm packages for angular projects which can lead to apps being broken -In general, harder to do changes across multiple apps and libraries as these are all in their own repository
If you are working on an enterprise project, it's recommended to organize your code in bigger parts like common service, UI components, etc. Thanks to the Nrwl team, we can accomplish this when working with monorepos by using Nx in our angular projects.
Lazy Loading
Thanks to lazy loading, we can improve the performance of our applications dramatically. Imagine you have a huge enterprise app and you don't want the app to take a long time to load. People will lose their minds and will stop using your app. Lazy loading will help us achieve a better performance of our app by loading our ngModules on demand. Some of the advantages of lazy loading your angular app are:
-High performance in bootstrap time on initial load -Modules are grouped according to their functionality (feature modules) -Smaller code bundles to download on initial load -Activate/download a code module by navigating to a route
If you want to learn more about lazy loading, take a look at the official Angular docs or this awesome article/tutorial.
https://angular.io/guide/lazy-loading-ngmodules
https://blog.bitsrc.io/boost-angulars-performance-by-lazy-loading-your-modules-ca7abd1e2304
Components
When creating an Angular app, I would suggest that you create 2 kinds of components: the smart and the dumb components.
Smart components are the only ones that have access to the services usually via DI and the source of data. The dumb components will be used as pure functions hence no side effects should be created by them (something comes in and something comes out).
Can I use smart/dumb components with state management?
Yes, you can apply the concept of smart and dumb components when doing state management with tools like NgRx.
When using smart and dumb components, you are going to come up with the need of using Input() and Output() to communicate between components. People have asked me: how far should I chain my components? I try to keep them to 1 level up and 1 level down. There may be special scenarios where you do 2 levels up and 2 levels down of component communication using Input() and Output() but please try to avoid this.
If you want to learn more about Smart and dumb components, take a look at this link: https://medium.com/@jtomaszewski/how-to-write-good-composable-and-pure-components-in-angular-2-1756945c0f5b
Component Libraries
When working on enterprise Angular application, it is common to make mistakes and stop following standards and company's practices. A way to achieve consistency in your applications is by using component libraries like:
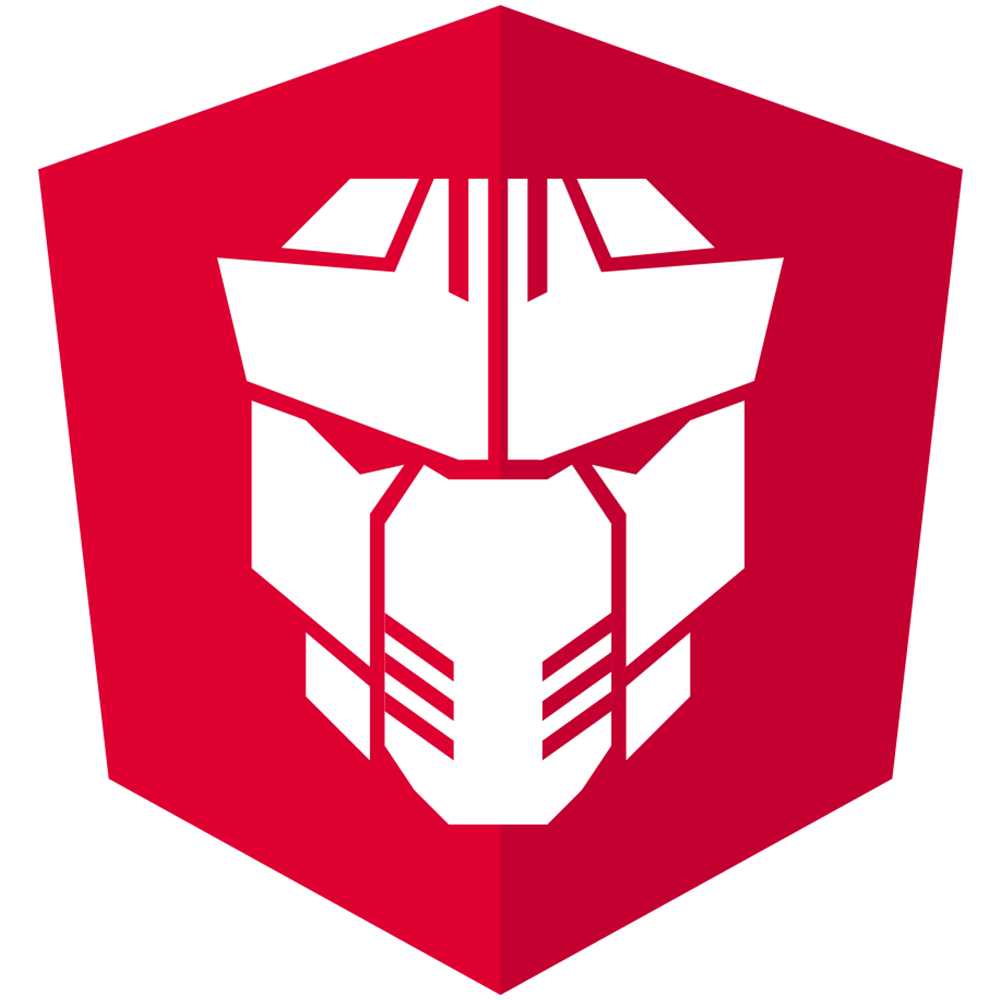
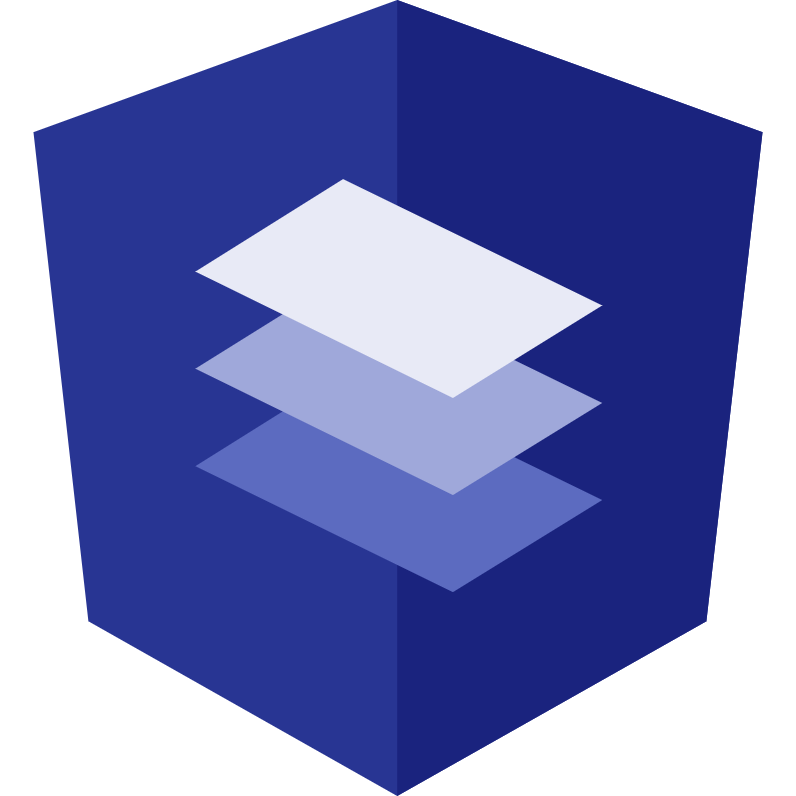
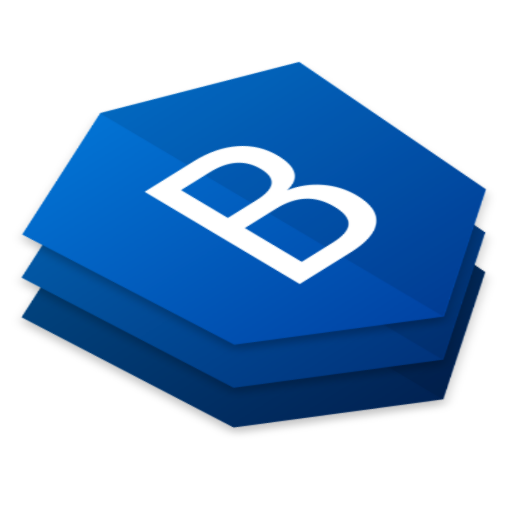
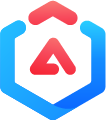
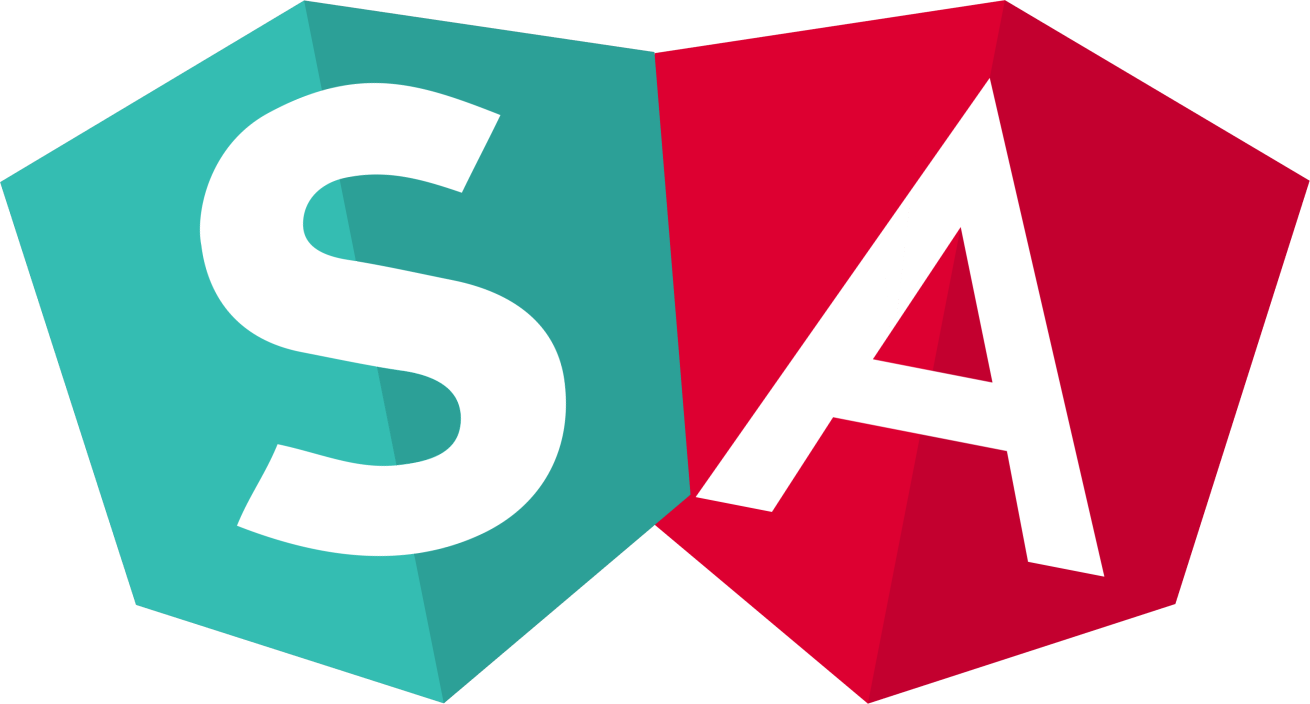
In my personal opinion, for an enterprise application, PrimeNG is the way to go. They have over 80 components that are easy to customize via CSS and multiple companies in the industry use PrimeNG which translates to good support by the community.
Design Systems
You may be wondering: what about custom components? Once again, keeping the standards of your company, the design, and the consistency in your application is very important. If your company doesn't have a designated UX/UI designer, taking a look at design systems will be a good place to start for keeping this consistency in your application's design.
Here are some great resources:
-Semantic UI: https://semantic-ui.com/ -PrimeNG: https://www.primefaces.org/designer/primeng -Material Design: https://material.io/design/ -Polaris Shopify: https://polaris.shopify.com/ -Ant Design: https://ant.design/
Reactive Programming with RxJS
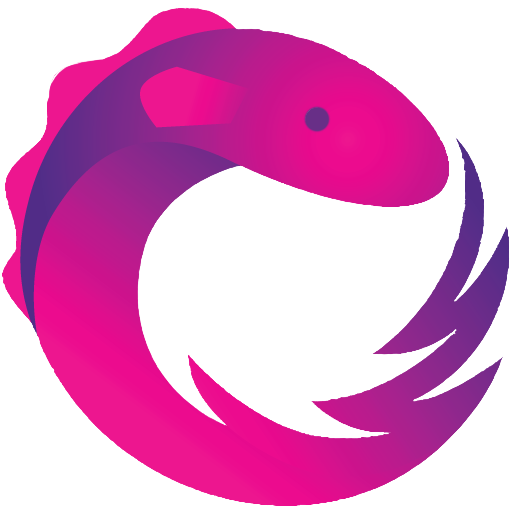
Time to start thinking reactively in Angular applications. We have an awesome library called RxJS that will help us achieve this.
When working with enterprise projects you are probably going to encounter getting data from multiple sources that depend on each other, or you will have to play a lot with multiple observable to achieve reactive programming. If this is the case, I'd suggest you take a declarative approach when using observables.
Benefits of a declarative approach: -Leverage the power of RxJs observables and operators -Effectively combine streams -Easily share observables -Readily react to user's action
To learn more about reactive programming, I'd suggest you take this course: https://www.pluralsight.com/courses/rxjs-angular-reactive-development
When working on the Angular project, if I have to consume promises I try to convert them to an observable. I do this because I want my app to be as reactive as possible. There are a few specific cases where I wouldn't convert promises to observables.
To learn more about Observables and Promises: https://medium.com/@mpodlasin/promises-vs-observables-4c123c51fe13
https://stackoverflow.com/questions/50269671/when-to-use-promise-over-observable
Compilation
Ivy is the new generation of the Angular compiler. Ivy will help you with your bundle size, re-build times, run-time performances, backward compatibility and more.
To learn more about Ivy: https://angular.io/guide/ivy https://blog.angularindepth.com/all-you-need-to-know-about-ivy-the-new-angular-engine-9cde471f42cf https://medium.com/js-imaginea/ivy-a-look-at-the-new-render-engine-for-angular-953bf3b4907a
Angular with Bazel
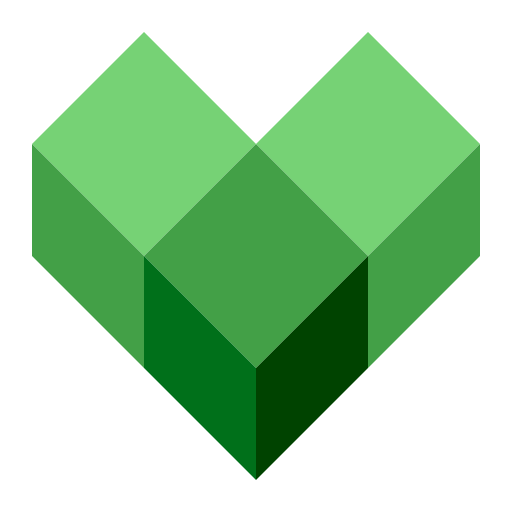
Thanks to the Google team, now we have this awesome tool called Bazel in our hands. Bazel allows us to build and test our backend and frontend with the same tool.
Bazel also helps your organization with continuous integration and it also helps with the build time. Bazel only builds what is necessary because Bazel uses a caching strategy where it only compiles what it has been changed or what is new.
learn more about bazel: https://blog.ninja-squad.com/2019/05/14/build-your-angular-application-with-bazel/
VS Tools
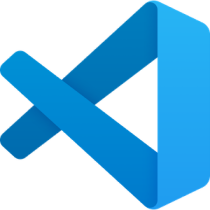
After several years in the industry, I have noticed that having the same environment settings can help us avoid merge conflicts and have a consistent codebase, especially when working with multiple developers across different teams.
The following plugins for VS Code will help your team develop your next Angular project.
-Angular Snippets (Version 8). -Angular Console. -Angular Schematics. -Prettier. -TS Lint. -Auto Rename Tag. -Highlight Matching Tag. -HTML Snippets. -IntelliSense for CSS class names in HTML. -JavaScript (ES6) code snippets. -RxJs Snippets. -Paste JSON as Code.
Got more?
If you have any other suggestions on how to help a team be successful in their enterprise project, put some comments and I will add them to the article.