At many points in your career, you will find yourself picking up a new framework, platform, language, or (most likely) some combination of all three. In my case, I recently found myself looking for a change of pace, and ended up switching from working in native iOS as a Mobile Engineer to the world of JavaScript as a Front-End Engineer. Specifically, I started out with Angular.
No matter how you slice it, learning something brand new can feel daunting — regardless of whether it is mid-career or otherwise. Luckily, Angular is extremely simple and quick to get set up . . . but getting an environment and project set up really is only a small part of the path to learning a platform, isn’t it? As I challenged myself to attempt to learn Angular as quickly as possible, I aimed to first break apart the approach by understanding:
- What Angular is
- What Angular's goal is
- What the fundamental pieces of Angular are
So, before we jump straight into some code, let's grasp these concepts first.
What is Angular?
First of all, Angular is not AngularJS. This was slightly confusing for me at first, and I made the mistake of mixing them up initially. Oops. At a macro level, Angular is a modern JavaScript framework that’s built on TypeScript, giving it access to static types, classes, and other elements provided by ES6. This allows code to organize and structure properly so it can be Object-Oriented, and allow for long-term maintenance at any scale, which will be important for the next fundamental concepts.
What does Angular aim to achieve?
Angular provides a platform to make single-page applications. I think it's important to point out the usage of the word platform, as Angular comes with quite a bit more to help us scale applications to any point we need. It ships with many integrated libraries (more on that in a bit), integration with numerous third-party libraries, and, maybe most importantly, the Angular CLI (command line interface), which gives us access to build, serve, test, and even ship our application. "Application" is also a very important distinction- that is, Angular (like many modern frameworks) aims to provide the tools to create responsive, smooth user experiences.
What are the essential building blocks of Angular?
To understand Angular, you have to understand what a Component is. But first, it may be easier to first visualize what makes an application: navbars, buttons, tables, table cells, and every other separately distinct building block you can visually see. In Angular, every single one of these is a Component, and everything else that exists is in service of these Components. For me, this is where it really started to click, since in iOS, the same fundamental principle exists: every single object on the screen must conform to UIView
, including any View Controller. Angular, of course, is no different — the root view of the app itself is also a Component.
Templates define the appearance of the Component via HTML. Angular extends the functionality of templates quite a bit through direct text interpolation, directives, and property/event binding. These, combined with CSS, can achieve essentially anything you need it to without having to entangle your Component's business logic with the presentation of said Component.
The final core piece of the Angular paradigm is dependency injection. Essentially, dependency injection helps keep your Components extremely light, modular, and maintainable. So, say you have a table that needs data from a request sent to an API endpoint. You could just have the inner workings of a server fetch right there all in the component, but you likely have multiple places all over your application where you'll be making API requests, and have to duplicate that code. Instead, Angular lets you define a Service object that can handle that API request, and return the data directly to your component. You don't even need to instantiate the Service since Angular will just automatically do that for you once you inject the dependency to keep things even cleaner.
Dependency injection, services, routing, etc. can feel daunting, but I think as long as you view everything else that isn't a Component solely existing to work in favor of Components, things become a lot clearer and you can start building apps the Angular way.
Speaking of, let's do a bit of project setup, take a look at the structure, build it, and add a component.
Prerequisties
Now that we know what Angular is, we can finally start our first project. A couple things we will need first:
- Visual Studio Code
- NodeJS and NPM with at least a basic proficiency with a terminal
- Some basic understanding of HTML and JavaScript/TypeScript
Creating and running your first project
With Node and NPM installed, we can install the Angular CLI with the following command:
npm install -g @angular/cli
Then we create our first project with:
ng new my-first-app
Note: The above command will have a couple prompts for you. Answer yes to add Angular routing, and the style sheet format is up to you (I chose SCSS). You can just press enter on both prompts for the default setup as well.
And finally, building and serving our new app locally:
cd my-first-app
ng serve
Open http://localhost:4200/
on your favorite browser to see what we just made. One note about ng serve
is its ability to pick up code changes and update live — no waiting for build times just to see very small changes here. Nice.
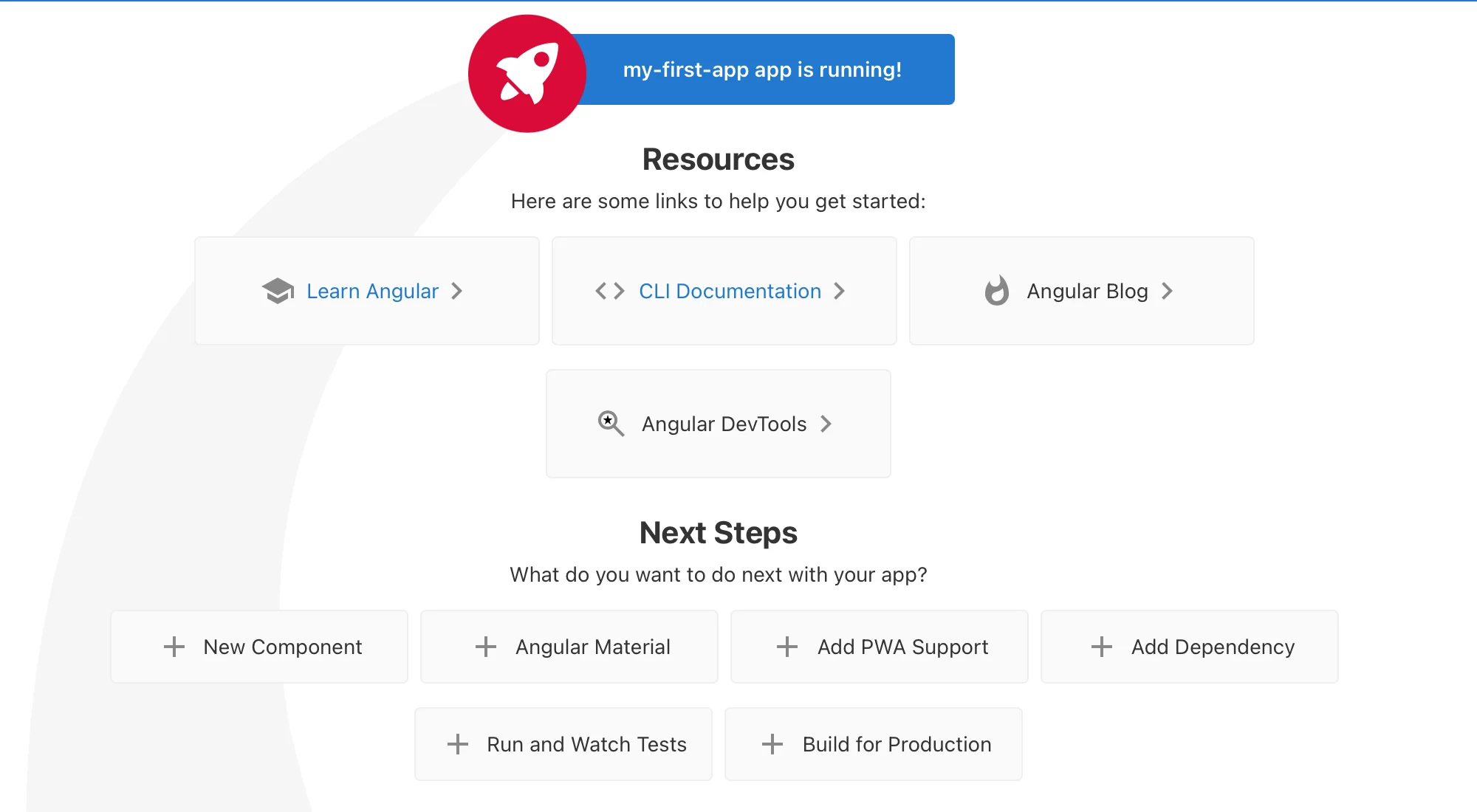
Project Structure
Of course, it's helpful to know what the CLI just built out for us. Looking at our project structure in VS Code, we can see quite a bit of config files set up in the root directory. Of course, these are all important, but for now, let's focus on getting familiar with a few:
angular.json
— This is the configuration file for CLI, so it knows how to build, serve, and test your app. Should be good to go right out of the box.package.json
— The file where the list of all your NPM dependencies live, thepackage-lock.json
specifies the versions for these files (for my iOS friends, this is very similar to thepodfile
orbuild.gradle
for my Android friends!). Whenever you install a Node package, the tool will automatically update these for you.node_modules
— With all the above dependencies installed, the.gitignore
should be ignoring this directory by default, but it's good to know everything is there.
Now that we're familiar with the housekeeping of the app, we can dig into the most important part: the code. In the src/app
directory, we can see our first module, component, and the files that make it up. Let's ignore those files for now, and create our very first Component.
Creating The First Component
Within your terminal, we'll run the following command:
ng generate component my-first-component
With that, CLI should have made a directory in src/app/my-first-component
and the following files:
my-first-component.component.ts
— This is the file where the data and logic needed to update your Component's Template live.my-first-component.component.html
— The associated Template for the Component, where the HTML is defined to actually render our Component.my-first-component.component.scss
— The associated style sheet for the Template, where styles scoped only to this Component are defined.my-first-component.component.spec.ts
— The very first unit test for the Component, automatically generated by CLI. How nice!
Within the component.ts
file, let's take special note of the following:
@Component({
selector: 'app-my-first-component',
templateUrl: './my-first-component.component.html',
styleUrls: ['./my-first-component.component.scss']
})
Here we can note the Template and style sheet URLs, but of particular note is the selector
, in our case called app-my-first-component
. This is the custom HTML tag we can now use anywhere our Component is imported in our project. The reason app
is prepended to the front of the name is because we defined this Component within the app module, but you can absolutely change the name if you want.
If we look back to the root app directory in src/app
(taking special note of the exact same file structure we just created in my-first-component
), let's open app.module.ts
. The CLI has already created a declaration for our Component in the NgModule
decorator, and imported MyFirstComponentComponent
automatically for us. Let's actually get our newly created Component on the screen somewhere.
Let's open app.component.html
. There's quite a bit of HTML already in here, but we can leave it mostly alone for now. Really, we can throw our Component just about anywhere, but let's search for the following:
<span>Welcome</span>
And replace it with:
<app-my-first-component></app-my-first-component>
Save your changes, and check your browser to see the following:

Now that we've got it up on the screen, let's do some slight modification to get data from the logic to the Template. First, add the following inside the class definition of my-first-component.component.ts
:
title = "Hello World!";
Then, inside my-first-component.component.html
, replace the Template definition with the following:
<p>{{ title }}</p>
The double bracket notation tells Angular to interpolate the value within, and dynamically pull the text defined in our class. Save both files, and you should see the following:

Neat, right?
Conclusion
Whenever someone asks me how easy it is to get started doing native iOS app development, it's easy for me to boast that you can start a project, throw something in Interface Builder, and run it — all within a few minutes' time. Now, I (and hopefully you) can easily say the exact same about Angular.
Of course, this is just a very small taste of what Angular has to offer, but hopefully instead of feeling initially lost in a sea of documentation, the relative simplicity of the platform will seem a lot more clear from the essence of what I described here from my journey of also having to learn Angular.
From here, I think it's valuable to look through the incredible official Angular docs, and I'd recommend running through some of their tutorials as well.