This is Part 2 of an ongoing series showing developers how to set up and deploy their own Shopify App! Find Part 1 here.
Today, we are going to continue our adventure! Last we left off, we made it through quite a bit, from setting up our Shopify App to retrieving customer orders. Though today's adventure will have us encounter retrieving fulfillment ids. This will be needed for our grand finale, which will be updating customer orders with tracking information. Now, if we have any new adventurers with us or you just need a recap of our last session, you can head over here.
Alternatively, if you just want the code from last time that can be found here. If you want to skip ahead and look at the code, it can be found here. With that all said, let us start retrieving those fulfillment ids!
We’re going start off by heading on over to our app/routes/app._index.jsx
file, and making changes to the loader function. Here, we need to retrieve our session, which we’ll do by adding:
const { session } = await authenticate.admin(request);
between our const orders and before our return json, and it should look like this afterward.
const orders = responseJson?.data?.fulfillmentOrders?.edges?.map(
(edge) => edge.node
) || [[]];
const { session } = await authenticate.admin(request);
return json({
orders: orders,
});
We’re going to need this to retrieve our fulfillment order information. With that, out of the way we’ll start by calling admin.rest.resources.FulfillmentOrder.all
to retrieve our fulfillment orders. This will require the session we added and the order IDs from the orders we retrieved above in our previous blog post. Here is a rough look at what the call will be.
const fulfillmentOrder = await admin.rest.resources.FulfillmentOrder.all({
session: session,
order_id: order.id,
});
We need to make a few changes though to get this up and running. First our order.id lives inside of an orders array so we’ll need to loop over this call. Second order.id also contains a bunch of unneeded information like gid://shopify/Order/
, so we’ll need to remove that in order to get the string of numbers we need. With those conditions outlined, we’ll go ahead and wrap it in a for loop, and use .replace to resolve the order.id issue, which gives us:
for (const { order } of orders) {
await admin.rest.resources.FulfillmentOrder.all({
session: session,
order_id: order.id.replace("gid://shopify/Order/", ""),
});
}
Now that we are able to loop over our call and have gotten the order IDs properly sorted, we still have a couple of issues. We need a way to use this data. So we’re going to set a variable to store the call, and then we’ll need a place to store the fulfillment ID(s). To store the fulfillment ID(s), we’ll create an array called fulfillmentIds.
const fulfillmentIds = [];
For the call, we’ll label it as fulfillmentOrder.
const fulfillmentOrder = await admin.rest.resources.FulfillmentOrder.all({
session: session,
order_id: order.id.replace("gid://shopify/Order/", ""),
});
We should now have something that looks like this:
const { session } = await authenticate.admin(request);
const fulfillmentIds = [];
for (const { order } of orders) {
const fulfillmentOrder = await admin.rest.resources.FulfillmentOrder.all({
session: session,
order_id: order.id.replace("gid://shopify/Order/", ""),
});
}
We’re now almost there, and we just need to figure out how we want to get the fulfillment ID(s) out of the fulfillmentOrder. To do this, we’ll map over fulfillmentOrder and check for the open status, and push the IDs found into our fulfillmentIds array.
for (const { order } of orders) {
const fulfillmentOrder = await admin.rest.resources.FulfillmentOrder.all({
session: session,
order_id: order.id.replace("gid://shopify/Order/", ""),
});
fulfillmentOrder.data.map(({ id, status }) => {
if (status === "open") fulfillmentIds.push({ id });
});
}
Awesome! Now we have our fulfillment ID(s)! We can now return our fulfillment ID(s) in our return json() section, which should look like this.
return json({
orders: orders,
fulfillmentIds: fulfillmentIds,
});
Our code should now look like this:
const { session } = await authenticate.admin(request);
const fulfillmentIds = [];
for (const { order } of orders) {
const fulfillmentOrder = await admin.rest.resources.FulfillmentOrder.all({
session: session,
order_id: order.id.replace("gid://shopify/Order/", ""),
});
fulfillmentOrder.data.map(({ id, status }) => {
if (status === "open") fulfillmentIds.push({ id });
});
}
return json({
orders: orders,
fulfillmentIds: fulfillmentIds,
});
We can now look at our terminal and see the fulfillmentIds array returning the fulfillment ID(s).
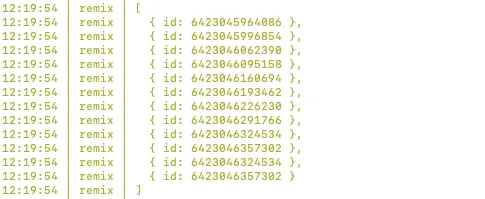
Conclusion
And there we have it! Part 2 is over and we can catch our breath. While it was a shorter adventure than the last one, a good chunk was still accomplished. We’re now set up with our fulfillment ID(s) to push on into the last part of the adventure, which will be updating customer orders with tracking information.